import
java.util.Scanner;
Output:
enter size of the table
10
press 1. insert 2. display 3. search 4. exit
1
enter key to be inserted
1
press 1. insert 2. display 3. search 4. exit
1
enter key to be inserted
11
press 1. insert 2. display 3. search 4. exit
1
enter key to be inserted
21
press 1. insert 2. display 3. search 4. exit
1
enter key to be inserted
31
press 1. insert 2. display 3. search 4. exit
2
no elements at index 0
elements at index 1 are 1->11->21->31->
no elements at index 2
no elements at index 3
no elements at index 4
no elements at index 5
no elements at index 6
no elements at index 7
no elements at index 8
no elements at index 9
press 1. insert 2. display 3. search 4. exit
3
enter key to be searched
1
element found in linked list 1
press 1. insert 2. display 3. search 4. exit
4
class SLLNode
{
int data;
SLLNode
next;
}
class
SeparateChaining
{
SLLNode
htable[];
int tableSize;
public void insert(int key)
{
int index=key%tableSize;
SLLNode
newnode=new SLLNode() ;
newnode.data=key;
newnode.next=null;
if(htable[index]==null)
htable[index]=newnode;
else
{
SLLNode
c = htable[index];
while(c.next != null)
c=c.next;
c.next=newnode;
}
}
public void display()
{
for(int i=0;i<tableSize;i++)
{
SLLNode
c=htable[i];
if(c == null)
System.out.println("no
elements at index " + i);
else
{
System.out.print("elements at index "+i +" are ");
while(c!=null)
{
System.out.print(c.data+"->");
c=c.next;
}
System.out.println();
}
}
}
public void search(int key)
{
int index=key%tableSize;
if(htable[index]==null)
System.out.println("element
not found");
else
{
SLLNode
c = htable[index];
while(c != null)
{
if(c.data == key)
{
System.out.println("element
found in linked list "+ index);
return;
}
c=c.next;
}
System.out.println("element
not found");
}
}
}
public class
SeparateChainingDemo
{
public static void main(String[] args)
{
SeparateChaining
ob=new
SeparateChaining();
Scanner
sc=new Scanner(System.in);
System.out.println("enter
size of the table");
ob.tableSize=sc.nextInt();
ob.htable=new SLLNode[ob.tableSize];
for(int i=0;i<ob.tableSize;i++)
ob.htable[i]=null;
while(true)
{
System.out.println("press 1.
insert 2. display 3. search 4. exit");
int choice=sc.nextInt();
switch(choice)
{
case 1:
System.out.println("enter
key to be inserted");
int key=sc.nextInt();
ob.insert(key);
break;
case 2:
ob.display();
break;
case 3:
System.out.println("enter
key to be searched");
key=sc.nextInt();
ob.search(key);
break;
default:
System.exit(0);
}
}
}
}
Output:
enter size of the table
10
press 1. insert 2. display 3. search 4. exit
1
enter key to be inserted
1
press 1. insert 2. display 3. search 4. exit
1
enter key to be inserted
11
press 1. insert 2. display 3. search 4. exit
1
enter key to be inserted
21
press 1. insert 2. display 3. search 4. exit
1
enter key to be inserted
31
press 1. insert 2. display 3. search 4. exit
2
no elements at index 0
elements at index 1 are 1->11->21->31->
no elements at index 2
no elements at index 3
no elements at index 4
no elements at index 5
no elements at index 6
no elements at index 7
no elements at index 8
no elements at index 9
press 1. insert 2. display 3. search 4. exit
3
enter key to be searched
1
element found in linked list 1
press 1. insert 2. display 3. search 4. exit
4
Algorithm to insert a value in hash table using separate chaining collision resolution technique
Hashtable is an array of pointers. All pointers are initialized to NULL
Step1: Read the value to be inserted, key
step 2: create a new node using new operator
step 3: Assign the value read to the data field of newnode (newnode.data =key)
step 4: compute the index
index = key % TABLE_SIZE
step 5: if head[ index] is NULL then
step 5.1: newnode becomes the only node in linked list or first node
step 6: else
step 6.1 : attach the newnode at the end of linked list as lastnode
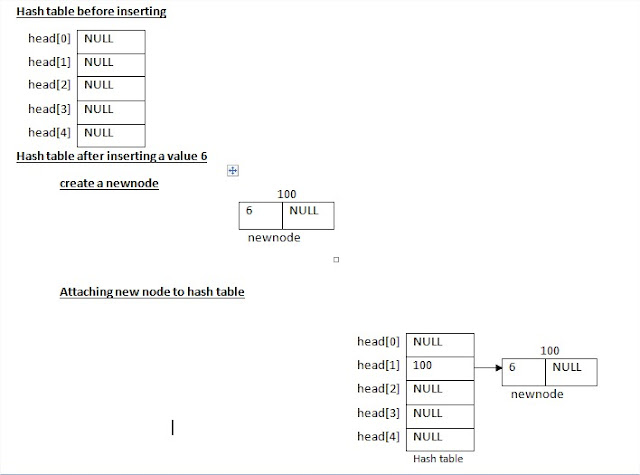
Best Article . Learn More about Technology
ReplyDeletehttps://www.mrlaboratory.info/